
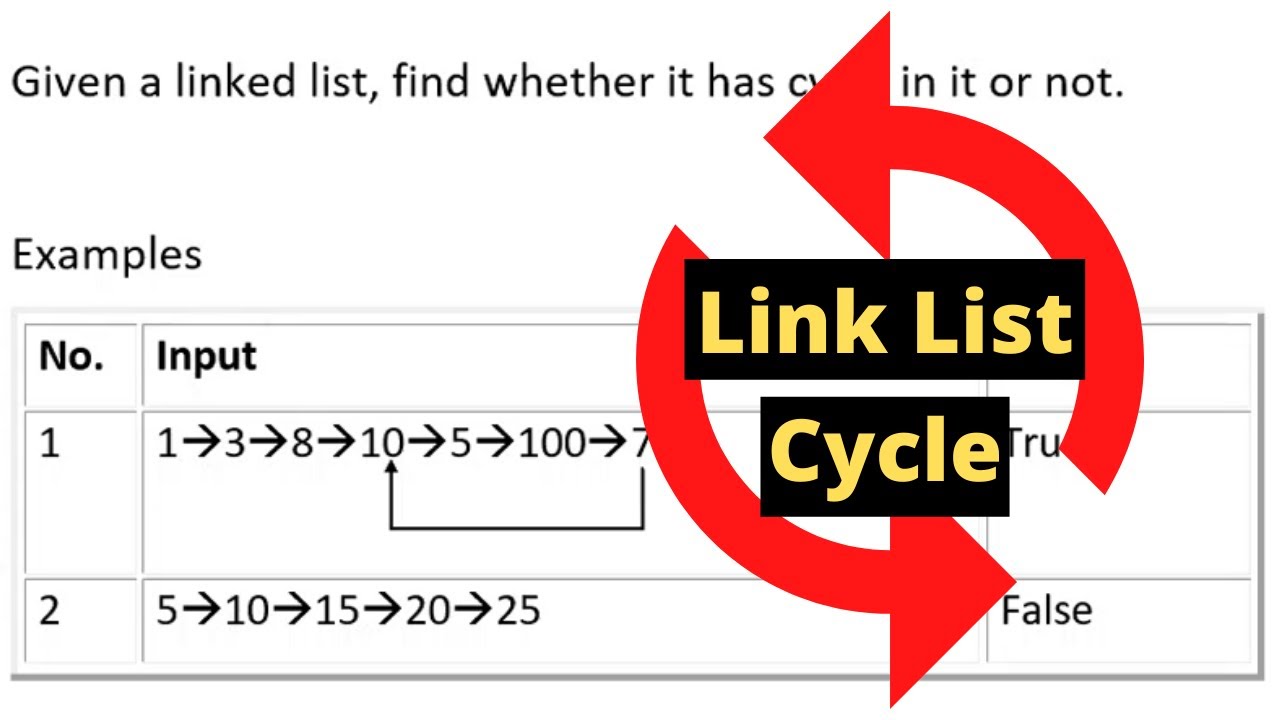
Right now you are removing the first occurrence - in future you might need to remove all matching occurrences, in which case you then have to rewrite the loop. You can't continue iteration after the remove unless you use the iterator's remove method.The collection could be, for example, a linked list (and in your case it is) whose remove method means searching for the object all over again, which search could have O(n) complexity.It is best to use an iterator and use it's remove method when searching for an object by iterating over a collection in order to remove it. I would treat this idiom similar to goto (or rather, labeled break/ continue): it may seem wrong at first, but when used wisely, it makes for a cleaner code.
#Removing a loop in a linked list stack overflow code
It may be best if you add a comment on the break, why it's absolutely necessary, etc, because if this code is later modified to continue iterating after a remove, it will fail. The iterator doesn't even get a chance to detect it. Here, after the remove (which qualifies as concurrent modification), you break out of the loop. In addition to that, we also keep top pointer to represent the top of the stack.
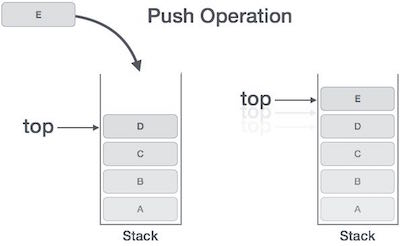
That's not the case here, so this shortcut is fine.Ī ConcurrentModificationException is checked and thrown by the iterator. A stack using a linked list is just a simple linked list with just restrictions that any element will be added and removed using push and pop respectively. To those who's saying that this will fail because you can't modify a collection in a foreach - this is true only if you want to keep iterating.
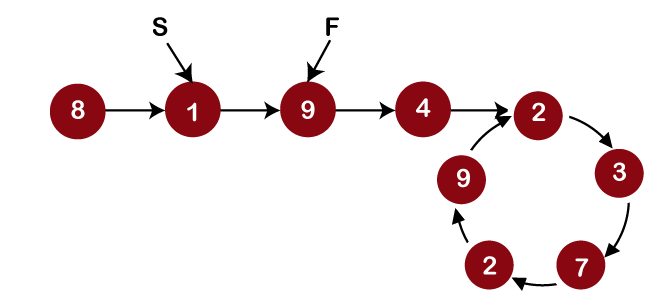
So yes, if you break out of the foreach after you remove, you'll be fine. If you want to delete both, you need to traverse the entire linked list by removing the return statements in the while loop and the initial check of the head node. Check if the head->next is null or not and if it is then check if the value in the head is the value you are searching for, remove it and return null or else do nothing. Because (S)'s speed is only one node at a time, it will meet (F) at (k) nodes from the loop's start - as in, (k) more steps before reaching the start, NOT (k) steps AFTER the start. Otherwise you'll get a ConcurrentModificationException, or in the more general case, undefined behavior. If the loop's start is NOT at the list's head, then by the time (S) enters the loop, (F) has had a head start of (k) nodes in the loop. If you want to keep iterating after a remove, though, you need to use an iterator. HOWEVER, in this case it's fine since you break out of the loop once you remove. Others have mentioned the valid point that normally this is not how you remove an object from a collection.
